本篇内容介绍了“Java泛型知识点有哪些”的有关知识,在实际案例的操作过程中,不少人都会遇到这样的困境,接下来就让小编带领大家学习一下如何处理这些情况吧!希望大家仔细阅读,能够学有所成!
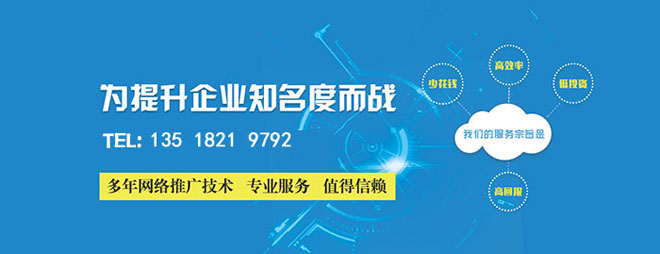
站在用户的角度思考问题,与客户深入沟通,找到润州网站设计与润州网站推广的解决方案,凭借多年的经验,让设计与互联网技术结合,创造个性化、用户体验好的作品,建站类型包括:成都网站设计、网站制作、企业官网、英文网站、手机端网站、网站推广、空间域名、网页空间、企业邮箱。业务覆盖润州地区。
一 什么是泛型
Java 泛型(generics)是 JDK 5 中引入的一个新特性, 泛型提供了编译时类型安全检测机制,该机制允许程序员在编译时检测到非法的类型。
简单理解就是:泛型指定编译时的类型,减少运行时由于对象类型不匹配引发的异常。其主要用途是提高我们的代码的复用率。
我们Java标准库中的ArrayList就是泛型使用的典型应用:
public class ArrayList extends AbstractList implements List, RandomAccess, Cloneable, java.io.Serializable { ...... public ArrayList(Collection c) { elementData = c.toArray(); if ((size = elementData.length) != 0) { // c.toArray might (incorrectly) not return Object[] (see 6260652) if (elementData.getClass() != Object[].class) elementData = Arrays.copyOf(elementData, size, Object[].class); } else { // replace with empty array. this.elementData = EMPTY_ELEMENTDATA; } } public void sort(Comparator c) { final int expectedModCount = modCount; Arrays.sort((E[]) elementData, 0, size, c); if (modCount != expectedModCount) { throw new ConcurrentModificationException(); } modCount++; } ..... public E get(int index) { rangeCheck(index); return elementData(index); } public boolean add(E e) { ensureCapacityInternal(size + 1); // Increments modCount!! elementData[size++] = e; return true; } }
二 extends和super通配符
在定义泛型类型Generic的时候,也可以使用extends通配符来限定T的类型:
public class Generic { ... }
现在,我们只能定义:
Generic p1 = null; Generic p2 = new Generic<>(1, 2); Generic p3 = null;
因为Number、Integer和Double都符合。
非Number类型将无法通过编译:
Generic p1 = null; // compile error! Generic